Sometimes, you have common routes that you want to reuse inside other resources and routes. For example, imagine that your application has two resources, photos
and posts
.
# config/routes.rb
resources :posts
resources :photos
Next, you decide that you want to allow users to add comments under both posts and photos. That means you'll want to nest comments under both resources as follows:
# config/routes.rb
resources :posts do
resources :comments
end
resources :photos do
resources :comments
end
This is simple enough. But, you can imagine this can get repetitive if you have few more 'commentable' resources, i.e. resources that can be commented.
To avoid this repetition, Rails lets you to declare these common routes (concerns) to reuse inside other resources and routes. For this, you'll use the concern
method as follows:
# config/routes.rb
concern :commentable do
resources :comments
end
resources :posts, concerns: :commentable
resources :photos, concerns: :commentable
# more than one concerns are allowed
resources :messages, concerns: [:commentable, :attachable, ...]
You can also use these concerns anywhere by calling the concerns
method.
# config/routes.rb
namespace :blog do
concerns :commentable
end
The underlying idea behind routing concerns is exactly similar to the concept of concerns in Rails: to group commonly used, related code together in one place.
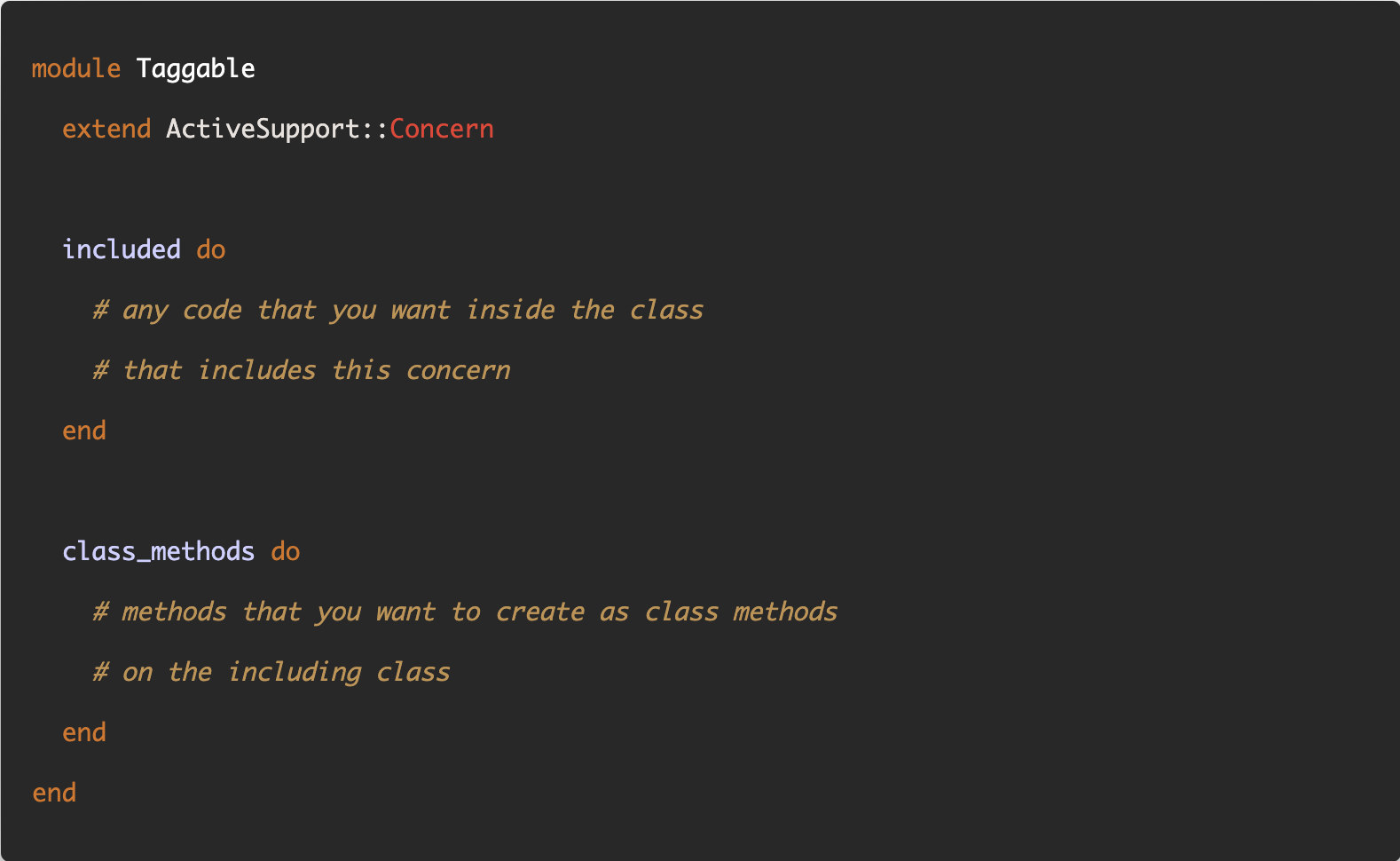
That's a wrap. I hope you found this article helpful and you learned something new.
As always, if you have any questions or feedback, didn't understand something, or found a mistake, please leave a comment below or send me an email. I reply to all emails I get from developers, and I look forward to hearing from you.
If you'd like to receive future articles directly in your email, please subscribe to my blog. Your email is respected, never shared, rented, sold or spammed. If you're already a subscriber, thank you.